import torch
import math
from torch.nn import L1Loss
from torch import nn
inputs = torch.tensor([1, 2, 3], dtype=torch.float)
targets = torch.tensor([1, 2, 5], dtype=torch.float)
inputs = torch.reshape(inputs, (1, 1, 1, 3))
targets = torch.reshape(targets, (1, 1, 1, 3))
# loss = L1Loss()
loss = L1Loss(reduction='sum')
result = loss(inputs, targets)
print(result)
loss_mse = nn.MSELoss()
result_mse = loss_mse(inputs, targets)
print(result_mse)
# 这个是用于目标分类的,3个目标的概率分别为:0.1,0.2,0.3,但是最终选择了0.2,也就是选择了1
# 这是输入
x = torch.tensor([0.1, 0.2, 0.3])
x = torch.reshape(x, (1, 3))
# 并且,此时y的batchSize就是1,不用去改变,这是输出
y = torch.tensor([1])
loss_cross = nn.CrossEntropyLoss()
result_cross = loss_cross(x, y)
print(result_cross)
print(-0.2 + math.log(math.exp(0.1)+math.exp(0.2)+math.exp(0.3), math.exp(1)))
Previous
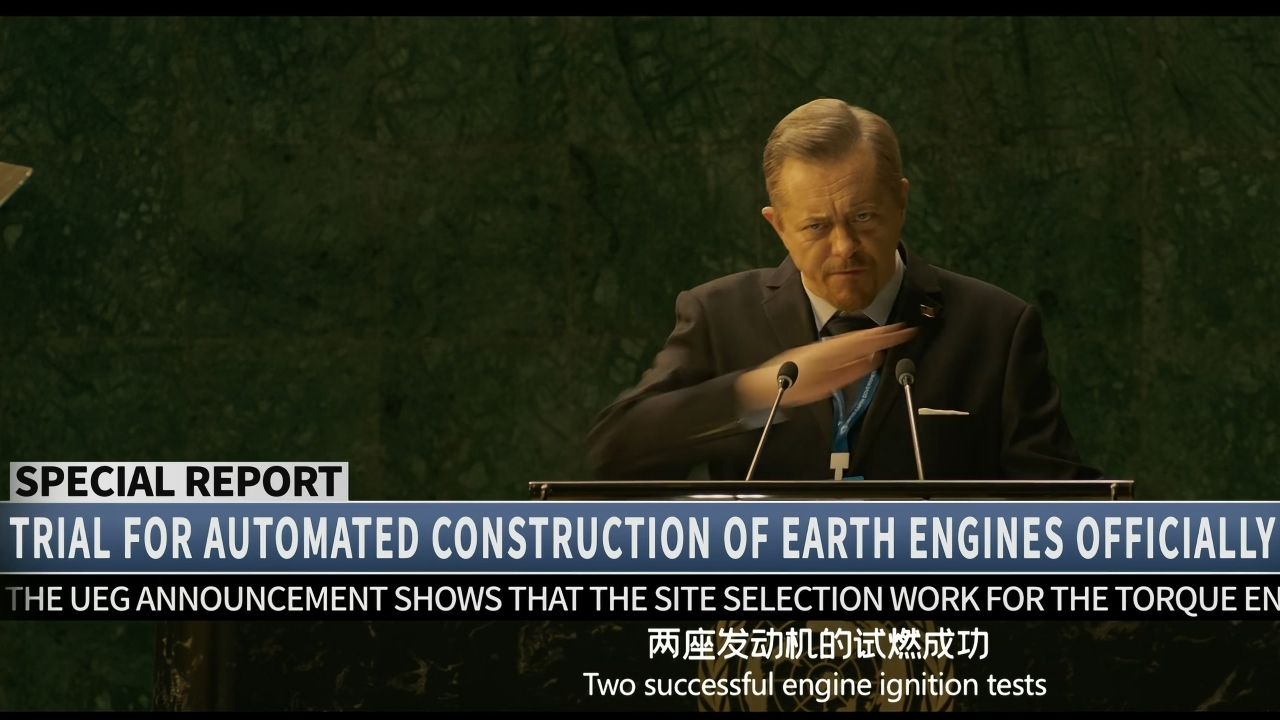
2021-11-16
Next
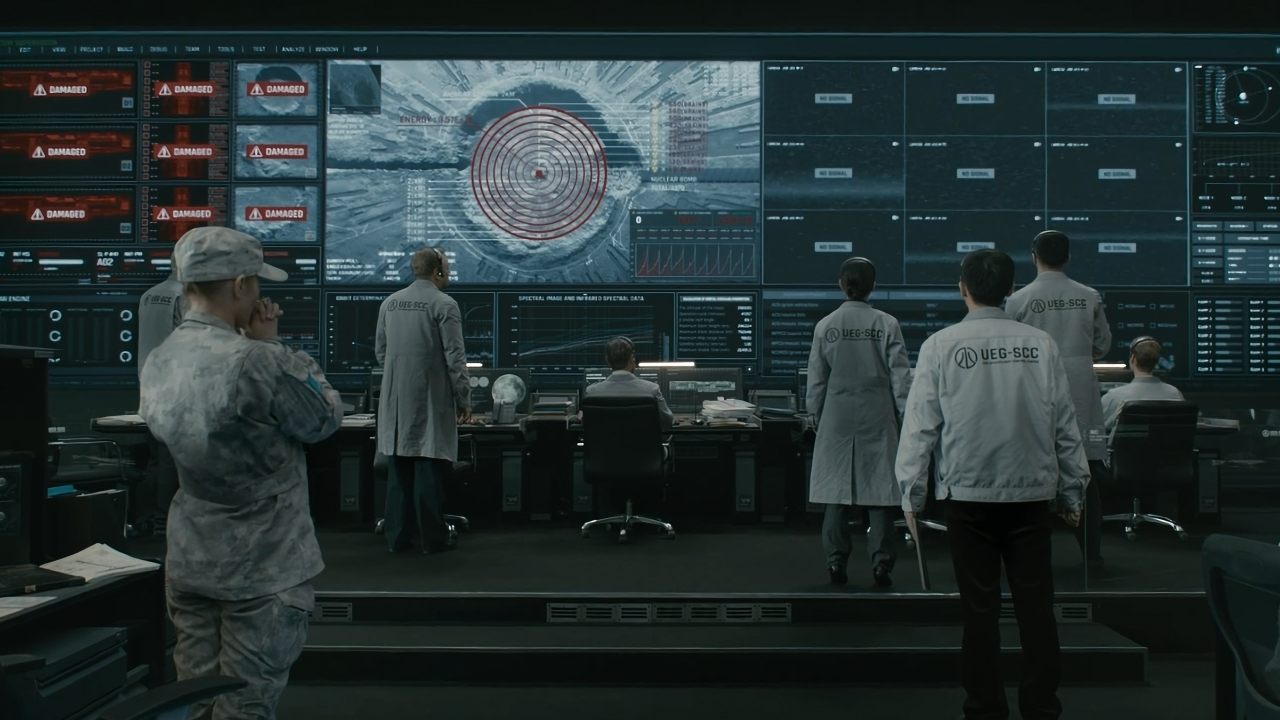
2021-11-14